Introduction
The way in which the input args are received by a rif plugin depends
on whether they are quoted or not quoted. For example, figure 1 shows the
"Rif Args" text field with three characters (a,b and c). The info message from the rif
(ParseArgs.cpp - listing 1) reports that only one arg was received by the plugin.
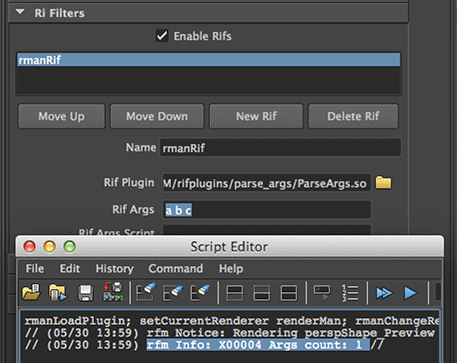
Figure 1
However, when the input characters are in quotations, as shown next,
the info message reports the arg count is 3.
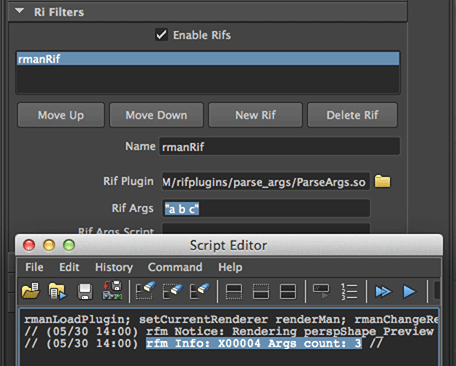
Figure 2
The arg counts for un-quoted and quoted inputs are the same when using Cutter's Rif Tool.
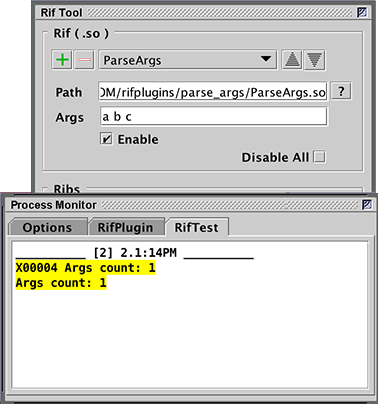
Figure 3
When testing how a rif should handle it's input args it is often more
convenient to use Cutter's Rif Tool than Maya for two reasons.
Firstly, once a rif has been used with Maya it is "stuck"
in memory - changing the cpp code and re-building the .so file will have no
effect in Maya. Secondly, rather than using "RixMessages" the standard output
stream (std::cout) can be used to output diagnostic messages.
|