Introduction
This tutorial presents a technique for generating a 32 bit image that is
suitable, once converted to a texture, for use as a displacement map that will produce a pattern of
high frequency bumps. The 32 bit image is derived from an orthographic rendering of a square
polygon lit by instances of a custom light source shader. The shader is implemented
such that its lighting distribution, figure 1, produces a gradation of grayscale values on
the square polygon that will cause the displacement map to produce hemi-spherical "bumps".
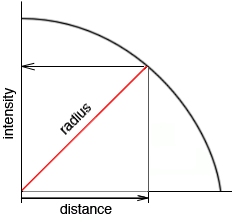
Figure 1
Light source - spherical drop-off profile
For example, the image shown in figure 2 was converted
to a texture and used as a map to displace the polygon shown in figure 3.
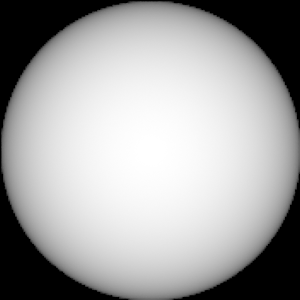
Figure 2
Orthographic image of a polygon
illuminated by a single custom light source.
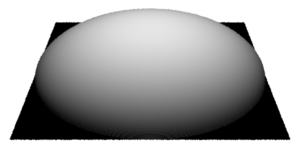
Figure 3
A polygon displaced by a texture map
from orthographic rendering of figure 2.
The tutorial uses a python script to write a pre-baked (archive) rib file
that references an arbitary number of light sources. For
example, figure 3 is an image of a polygon lit by 1600 lights distributed in a
regular grid. Figure 4 shows the effect of using the image as a displacement map
on a nurbs sphere. Despite the very high frequency of the bumps at the pole of
the sphere the pattern displays very little aliasing - a benefit derived from
the use of a texture map.
|